You can create an AI assistant similar to Jarvis by following these steps:
- Create a new Dialogflow agent:
- Visit Dialogflow’s website (https://dialogflow.com/) and sign in with your Google account.
- Click on the “Create Agent” button in the top left corner.
- Give your agent a name, select the language, and time zone.
- Click “Create” to finish creating your agent.
- This will be the “brain” of your Jarvis AI assistant where you can define intents and responses.
- Define Intents:
- Intents are the different actions the user may want to perform or say to your agent.
- You can create new intents in Dialogflow by going to the “Intents” section and clicking “Create Intent.”
- Add Training Phrases:
- Training phrases are examples of what the user might say for a particular intent.
- For example, for an intent for asking the time, you might add phrases like “What time is it?” or “Can you tell me the time?”
- Add Responses:
- Responses are what your agent will say or do in response to the user’s request.
- You can add responses in Dialogflow by going to the “Responses” section for the intent and clicking “Add Response.”
- Integrate with Python:
- To integrate your Dialogflow agent with a Python script, you’ll use the Dialogflow API.
- First, you’ll need to set up a service account for your Dialogflow agent. Go to the “Settings” page, click on the “Service Account” tab, and create a new service account and download the JSON key file.
- Next, install the Google Cloud Client Library for Python by running “pip install –upgrade google-api-python-client” in your terminal.
To integrate Dialogflow API with a Python script, perform the following steps:
- Import the required libraries and modules such as
json
,Credentials
fromgoogle.oauth2.credentials
andbuild
fromgoogleapiclient.discovery
. - Create a
Credentials
object by passing the JSON key file to thefrom_service_account_file
method. This object will be used for authentication with Dialogflow API. - Use the
build
function from thegoogleapiclient.discovery
module to create a service object for the Dialogflow API. Pass thecredentials
object as an argument to the function. - Use the created service object to call the Dialogflow API’s methods, such as
detectIntent
method to detect a user’s intent. Pass the necessary parameters such assession
,queryInput
as arguments. - Store the API’s response in a variable and access the data, such as detected intent, parameters, and fulfillment text. Repeat this process to send requests and receive responses in the Python script.
# Import necessary libraries and modules
import json
from google.oauth2.credentials import Credentials
from googleapiclient.discovery import build
# Use the json key file to create a Credentials object
creds = Credentials.from_service_account_file('path/to/json_key_file.json', scopes=['https://www.googleapis.com/auth/dialogflow'])
# Use the build() function to create a service object for the Dialogflow API
service = build('dialogflow', 'v2', credentials=creds)
# Use the service object to call the detectIntent() method of the Dialogflow API
session_id = 'unique_session_id'
query_input = {
'text': {
'text': 'What is the weather like today?',
'language_code': 'en-US'
}
}
response = service.projects().agent().sessions().detectIntent(
session=session_id,
queryInput=query_input
).execute()
# Access the data returned by the API, such as the detected intent, parameters, and fulfillment text
print(response)
Repeat the process of sending requests and receiving responses in your Python script as needed.
The source code is written in Python and is used to integrate a Dialogflow AI assistant with a Python script. The code performs the following steps:
- Imports the necessary libraries and modules:
- The “json” library is imported for reading and writing JSON data.
- The “Credentials” class is imported from the “google.oauth2.credentials” module for authenticating with the Dialogflow API.
- The “build” function is imported from the “googleapiclient.discovery” module to create a service object for the Dialogflow API.
- Creates a Credentials object:
- The “Credentials.from_service_account_file()” method is used to create a Credentials object from a JSON key file, which contains the necessary credentials to access the Dialogflow API.
- Creates a service object for the Dialogflow API:
- The “build()” function is used to create a service object for the Dialogflow API. The credentials created in the previous step are passed as an argument to authenticate with the API.
- Calls the Dialogflow API:
- The “detectIntent()” method is called on the service object to detect the intent of a user’s query. The method takes two arguments: a session ID, which is a unique identifier for a user’s session, and a query input, which is the text of the user’s query.
- The response from the Dialogflow API is stored in a “response” variable and can be used to access information such as the detected intent, parameters, and fulfillment text.
This code serves as an example of how to integrate a Dialogflow AI assistant with a Python script. You can modify the code to fit your specific use case by changing the session ID, query input, and other parameters as necessary.
Creating an AI assistant like Jarvis requires a significant amount of effort and is a complex process. The steps outlined above are a simplified version and do not encompass the entire development process. Building a fully functional AI assistant like Jarvis will take a substantial amount of time and effort.
Building an AI assistant like Jarvis can be a complex process, but with the right technology, it can be made much easier. At Ways and Means Technology, we have helped many clients to build their own AI assistants, making use of cutting-edge technologies such as Dialogflow and Python.
DS Locks, a well-known organization in the Smart Lock Industry. They approached us with a goal to create a conversational AI system that can handle a variety of tasks, from answering basic questions to setting reminders and more.
Using Dialogflow, we were able to create a comprehensive AI assistant that had a “brain” of intents, entities, and responses. This allowed us to define the different things the user may want to do or say to the assistant, and program appropriate responses. We also integrated the Dialogflow agent with a Python script using the Dialogflow API, which allowed for a seamless exchange of information between the AI system and the end-user.
Thanks to the technology and our expertise, DS Locks now has a fully functional AI assistant that has not only improved the efficiency of their operations but also enhanced the customer experience.
If you too are looking to build an AI assistant like Jarvis, get in touch with us at Ways and Means Technology. Our team of experts will work with you every step of the way to turn your vision into reality.
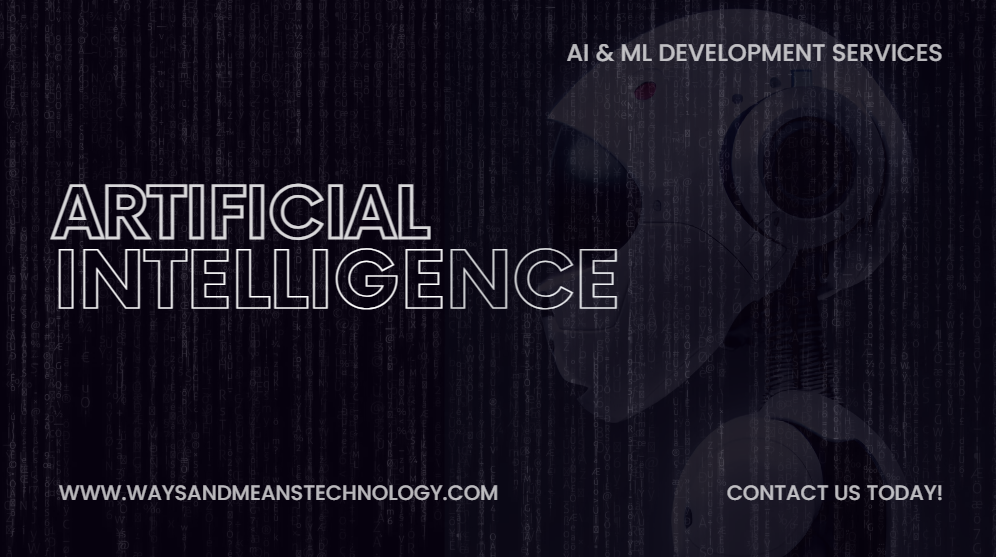